SOLID Principles - Interface Segregation Principle
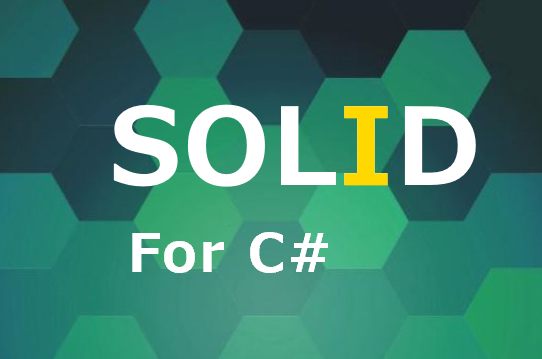
The fourth of the SOLID Principles to cover is the letter I which is the Interface Segregation Principle (ISP).
What is the Interface Segregation Principle (ISP)?
The Interface Segregation Principle (ISP) states that “Clients should not be forced to depend upon interfaces that they do not use". A simple interpretation of this statment would be that you should never force a class to implement methods of an interface it does not need.
Think of it another way, it’s better to create multiple smaller interfaces as opposed to creating one large complex “fat” interface.
An Example
Let’s pretend we are building out some functionality to load items into vehicles to ship.
interface IShippingVehicle
{
void LoadBox(string box);
void LoadCrate(string crate);
void LoadShippingContainer(string shippingContainer);
}
class DeliveryTruck : IShippingVehicle
{
public void LoadBox(string box)
{
// load box into truck
}
public void LoadCrate(string crate)
{
// load crate into truck
}
public void LoadShippingContainer(string shippingContainer)
{
throw new NotImplementedException();
}
}
class CargoShip : IShippingVehicle
{
public void LoadBox(string box)
{
throw new NotImplementedException();
}
public void LoadCrate(string crate)
{
// load crate into ship
}
public void LoadShippingContainer(string shippingContainer)
{
// load shipping container into ship
}
}
Ok, this may be a tad silly example but lets look at the issue that we have.
Both the DeliveryTruck and CargoShip are being forced to impliment functionality that they can not support. Wouldn’t it be nicer to only be required to impliment what they actually support!
Let’s give it another try this time by splitting up the interfaces into smaller pieces so the classes can choose what they want to support.
interface IBoxShippingVehicle
{
void LoadBox(string box);
}
interface ICrateShippingVehicle
{
void LoadCrate(string crate);
}
interface IShippingContainerShippingVehicle {
void LoadShippingContainer(string shippingContainer);
}
class DeliveryTruck : IBoxShippingVehicle, ICrateShippingVehicle
{
public void LoadBox(string box)
{
// load box into truck
}
public void LoadCrate(string crate)
{
// load crate into truck
}
}
class EverGivenCargoShip : ICrateShippingVehicle, IShippingContainerShippingVehicle
{
public void LoadCrate(string crate)
{
// load crate into ship
}
public void LoadShippingContainer(string shippingContainer)
{
// load shipping container into ship
}
}
There we go! Much cleaner and now we are not violating the Interface Segregation Principle (ISP).