SOLID Principles - Open-Closed Principle
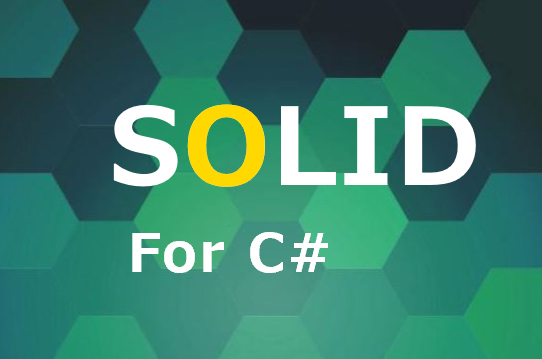
The second of the SOLID Principles to cover is the letter O which is the Open-Closed Principle (OCP).
What is the Open-Closed Principle (OCP)?
The Open-Closed Principle (OCP) states that “You should be able to extend a classes behavior, without modifying".
Let’s break that down as it’s really two parts of the whole.
First is the Open part. What this means is that when we design classes so that they can easily be added to when we add new functionality.
Secondly we have the Closed part. Here we are being informed that it’s a best practice to not modify existing code unless you’re fixing a bug.
Now you probabbly read that and said to yourself “Hey wait a darn minute! That makes no sense!”
Don’t worry it will shortly.
An Example
Let’s go back to our Order class from the first principle.
public class Order
{
public ICollection<Item> Items { get; set; }
public Customer Customer { get; set; }
public OrderRepository orderRepository = new OrderRepository();
public Logger Logger = new Logger();
public void PlaceOrder()
{
try
{
orderRepository.AddOrder(this);
}
catch (Exception ex)
{
Logger.Log(ex);
}
}
}
You’ve just been told you need to add support for the creation of recurring orders.
Well you could start adding the new functionality to the existing class like this.
public class Order
{
public ICollection<Item> Items { get; set; }
public Customer Customer { get; set; }
public bool IsRecurring { get; set; }
public CalendarService calendarService = new CalendarService();
public OrderRepository orderRepository = new OrderRepository();
public Logger Logger = new Logger();
public void PlaceOrder()
{
try
{
if (this.IsRecurring) {
calendarService.ScheduleRecurringOrder(this);
}
else
{
orderRepository.AddOrder(this);
}
}
catch (Exception ex)
{
Logger.Log(ex);
}
}
}
But wait a minute! The Open-Closed Principle (OCP) tells us that classes should be Closed to modification.
This is where the power of polymorphism and class inherentance comes in. We will create a brand new class that is built on top of the original order class without having to touch the original class.
public class RecurringOrder : Order
{
public CalendarService calendarService = new CalendarService();
public new void PlaceOrder()
{
try
{
calendarService.ScheduleRecurringOrder(this);
}
catch (Exception ex)
{
Logger.Log(ex);
}
}
}
Now this is better! We kept the original Order class closed from modification but it was open for extension by creation of the derived class.